装饰器
类装饰器
const doc:ClassDecorator = (target:any) =>{
console.log(target)
target.prototype.name = "meowrain";
}
@doc
class Meowrain {
constructor(){
}
}
const meow:any = new Meowrain();
console.log(meow.name)
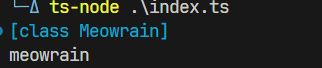
属性装饰器
const doc: PropertyDecorator = (target: any, key: string | symbol) => {
console.log(target, key)
}
class Meowrain {
@doc
public name: string;
constructor() {
this.name = "meow"
}
}
const meow: any = new Meowrain();
console.log(meow.name)
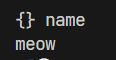
方法装饰器
const doc: MethodDecorator = (target:any,key:string|symbol,descriptor:any) =>{
console.log(target,key,descriptor);
}
class Xiaoman {
public name: string;
constructor(name:string) {
this.name = name;
}
@doc
getName(name:string,age:number) {}
}
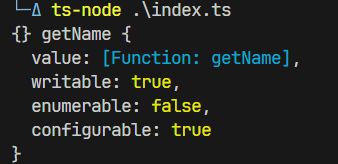
参数装饰器
const doc: ParameterDecorator = (target: any, key: string | symbol | undefined, index: number) => {
console.log(target, key, index);
};
class Meowrain {
public name: string;
constructor() {
this.name = "meowrian";
}
getName(@doc name: string, @doc age: number) {
console.log(name, age)
}
}

装饰器实现nestjs底层GET
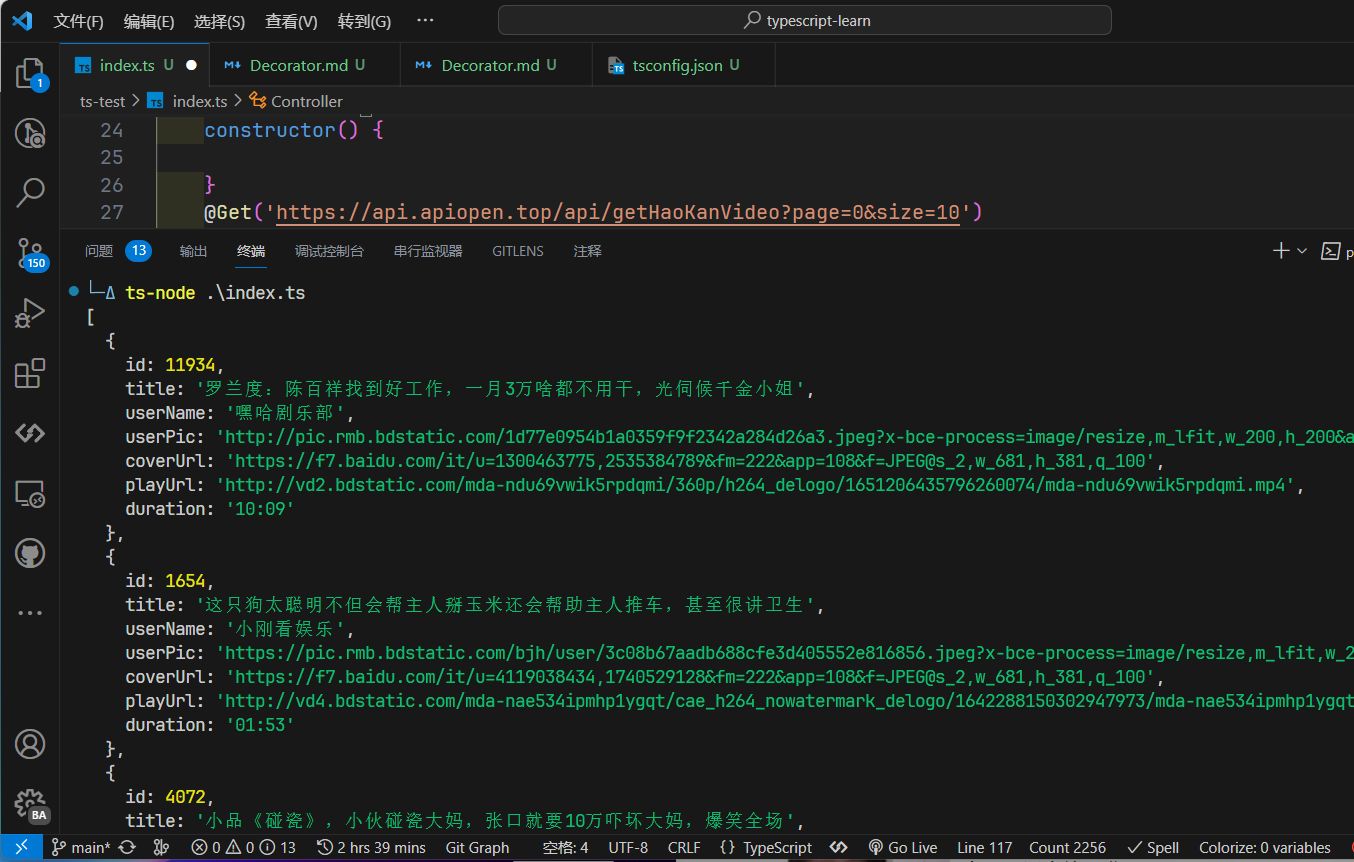
import axios from "axios"
const Get = (url: string) => {
return (target: any, key: any, descriptor: any) => {
const fnc = descriptor.value;
const AxiosGet = async (url: string) => {
try {
const res = await axios.get(url);
return fnc(res, {
status: 200,
success: true
});
} catch (e) {
return fnc(e, {
status: 500,
success: false
});
}
};
AxiosGet(url);
}
}
class Controller {
constructor() {
}
@Get('https://api.apiopen.top/api/getHaoKanVideo?page=0&size=10')
getList(res:any,status: number) {
console.log(res.data.result.list,status)
}
}